First published: Oct. 8, 2023 | Permalink | RSS
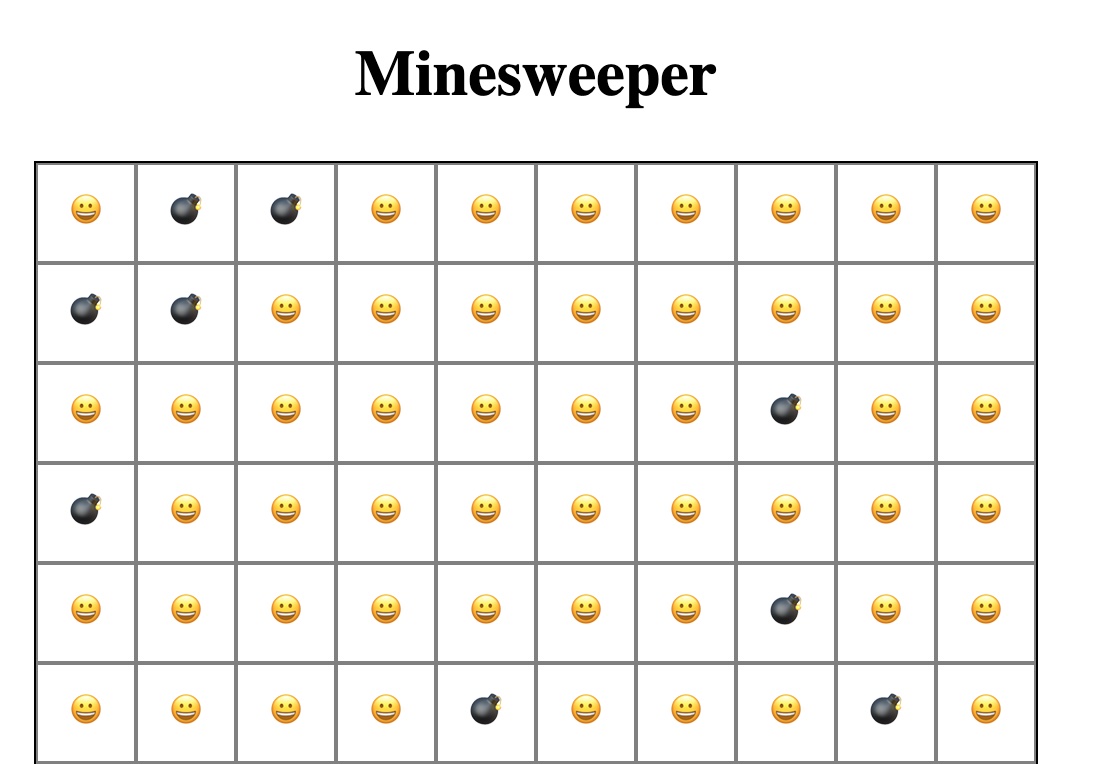
Our partially done Minesweeper game with randomly placed bombs and safe squares
Overview
Let’s make minesweeper with just native web tools! Using only native web components we can build this classic video game using some loops, array methods, and recursion. Specifically we'll be using HTML5, CSS3 with Flexbox, and ES6 JavaScript.
Prerequisites
To make this project you will need:
- A code editor (I’ll be using VS Code)
- A web browser
- A basic understanding of HTML, JavaScript, and CSS
How to make Minesweeper part 1:
Make your files
Start a project folder called “minesweeper” and in it create 3 files:
- index.html
- style.css
- app.js
Note: If you don’t already navigate your machine and manage your code files in the terminal or shell I recommend it! To do the above:
# Make your folder and change directories to it
mkdir minesweeper
cd minesweeper
# Create the files
touch index.html style.css app.js
Add your boilerplate code
Open all three files into your editor of choice. In index.html start with the boilerplate code (in VS Code you can type !
and click the first option, this fills it out as a snippet):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Title</title>
</head>
<body>
</body>
</html>
In the <body>
section, add: <h1>Minesweeper</h1>
and change the text in the <title>
tag to also read Minesweeper.
Open index.html in your browser. You can drag and drop the file into the browser or use open index.html
in your terminal or even use the file://
protocol if you’re feeling fancy.
This isn’t much of a website yet, and certainly not a game or stylish. But to get either of those qualities we need to connect our app.js
and style.css
files. To do so we need two lines in the head section of the html:
<link rel="stylesheet" href="style.css">
<script src="app.js"></script>
Then in the style.css
file we will need the following:
h1 {
color: pink;
}
It doesn't have to be pink, but it does help to have something stand out pretty dramatically. Now in our app.js
file let's add:
console.log("This is connected");
Note: I want to establish a pattern here that whenever we add new code to our files, we should test it. In this case, we’ll refresh the browser to see what we changed. So each time we make a change I’ll refer to this step as “refresh and check”. Also, each section in this is designed to be its own commit (and the titles of the sections work as good commit messages).
When we refresh and check, we should see our title on a pink background. To see the console.log message, we’ll have to right-click, and choose “inspect” from the context menu. If it’s your first time opening the inspector, welcome! Most browsers open by default to to the Elements inspector tab to let you see the html and css as it’s rendered on the page. And we’ll come back to that later. For now, let’s choose the Console tab and we should see our message!

Add the board and put stuff in it
A key part of the minesweeper game is a board of squares on a grid. So let’s add a <div>
element with the id: “board” (this will look like <div id="board"></div>
). A div by itself will not really do much. So we'll add our squares with JavaScript.
There’s no prescriptive size that our “minefield” should be, and in fact many implementations use many different sizes, but a 10x10 grid has an appeal for both easy numbers and a nice size for playing.
So to make a 10x10 board we will need to make 100 squares. But rather than using 10 and 100, let’s use variables like width
and boardSize
so we can make this easier to work on later. So in our app.js
file we'll replace our console.log
line with:
const width = 10;
const boardSize = width * width;
const board = document.getElementById("board");
for (let i = 0; i < 100; i++) {
const square = document.createElement("p");
square.innerHTML = `${i + 1}`;
board.appendChild(square);
}
Let's check and refresh. Here we run into an interesting bit of trouble, there should be 100 numbers on the screen and there are none and an error is in the console telling us no element exists with an id of "board." Code in HTML, CSS, and JS is read by the browser from top to bottom. The HTML file asks for the <link>
to the style sheet in the <head>
and then asks for the <script>
containing the Javascript. Then once the <head>
is read and rendered, the browser executes the <body>
code.
This means that in this case the <div>
with the id of "board" hasn't been rendered yet when the JavaScript is executed. There is a window.load
event we can ask for, but another way to handle this is to move the <script>
tag to the bottom of the <body>
section. This way the Javascript is only run once the whole page is rendered.
Refresh and check again and we have 100 numbers in our "board".

Style the board to be square
Now we have 100 numbers in a weirdly long list, but that’s not much of a board. For a moment we will need to write some CSS.
Note: For a lot of engineers, CSS can be very daunting, especially where page layout is concerned. And yes there are many component libraries that will handle this for us, but those libraries compile or otherwise eventually render CSS. Therefore, knowing how CSS works natively, it will help us to diagnose and solve design and UX issues because they come up with libraries too.
We will worry about the aesthetic style of the game later, for now we want to get the board to be functional. And for now, we only have a few elements on the page to worry about first. We have the board itself and 100 <p>
elements within it.
The board is a <div>
with an id
of "board" so we can easily grab that with #board
in our CSS. We'll set an arbitrary width (ideally one that will make math easy later), center it, and add a border so we can see the board.
#board {
width: 500px;
margin: 0 auto;
border: 1px solid black;
}
A refresh and check shows us a square with a border at the center of our page, but the numbers are still falling down the page. There's a few ways to get our elements to sit in a grid in the board, and my go-to is Flexbox, which is natively supported in CSS3. In this case it's appropriate since we have one array that we are arranging as a 2-dimensional grid and flexbox will treat them that way as well.
Note: Flexbox Froggy is a great way to learn how to use the basics of Flexbox and CSS Tricks has an excellent reference guide.
Let's add the following lines to our #board
CSS block to use Flexbox and display its children (our squares) as rows that wrap to the next row when they run out of horizontal space.
display: flex;
flex-flow: row wrap;
We still need to style the numbers to get them to fit nicely since their defaults will change depending on what text is in them. We will want to give them a fixed width and height, a border so we can see them for now, and center the text in each square.
Above, we (completely arbitrarily) chose 500px
as our board width and a game board size of 10 squares by 10 squares. So each square should be 50px
, but if we just set height and width to be 50px
, the squares wouldn't fit. HTML elements render width as the content (in this case: text) width. Padding, border, and margin will add more size to the box and will not fit on our grid.
We don't want any margin, since each square will sit directly against its neighbors. We also don't want padding since we're just centering with text-align
. However, we do want a border so we can see where the boxes are.
So, we will start our squares with the following styles: A width and height of 48px
to leave room for the 1px
of border on each side (e.g. for width, the left and right borders both add to the overall width).
#board p {
width: 48px;
height: 48px;
margin: 0;
padding: 0;
line-height: 48px;
text-align: center;
border: 1px solid gray;
}
A refresh and check should now show us a board with 100 squares arranged in a nice grid.

Make the squares clickable
Now that we have a grid, let's make it interactive! This is typically an expectation of any video game. The document
API has an addEventListener
method that is incredibly useful for this. The best place to add these event listeners will be as we make each square. So let's make our loop that creates the squares look like this.
for (let i = 0; i < 100; i++) {
const clickedClass = "clicked";
const square = document.createElement("p");
square.innerHTML = `${i + 1}`;
square.addEventListener("click", (e) => {
if (!square.classList.contains(clickedClass)) {
square.classList.add(clickedClass);
}
});
board.appendChild(square);
}
Note: clickedClass
may be extraneous for now, but we wanted to use the string "clicked"
more than once and it often helps to set that with a constant to ensure they match (and to make changing it easier later). I have had plenty of projects get stalled for a little too long trying to find a typo in a string.
And now that we are adding a "clicked" class to each square, let's change the color to see if our event handling works by adding this to our CSS:
.clicked {
background-color: green;
}
Refresh and check and click around the board. Any unclicked squares will change color now! However, as it's written above, we are only adding a class if it's not already there. If you'd like it to change the color back on the second click instead, try square.classList.toggle(clickedClass)

Refactor board to build from array of values
Now that we have a grid of 100 numbers ready to be built and clicked, let's add some mines to our minefield! In our javascript, let's create an array of 100 elements, with 20 bombs and 80 empties.
But what data should we put in this array? We have a lot of options, but since we are developing this as a webpage, adding classes to our square elements gives us an easy way to check if that square is a bomb (and then style it) by checking square.classList.contains()
.
Note: For a savvy game player, this does expose the locations via the inspector if a player wanted to cheat. If we were building something that needed to be competitive, a better choice would be to refer back to our array and check the value there. While less likely, that savvy player could still have access to that array via the console. In that case, a backend server would probably be necessary to keep things competitive. This is certainly outside our scope here, but it's always worth thinking about security especially when practicing.
So if we are going to use classes on each square, an array of strings would be a good choice. The strings in this case will be "bomb" and "valid", as in: There is either a bomb in this square or this square is a valid place to click. Booleans like true
and false
could also work, however, with enough distance it may become muddy wondering if it's true
this is a bomb or true
that we can safely click it.
Note: In this case I've chosen the word "bomb" instead of "mine" since "mine" has a few common meanings (place to dig for ores, belonging to me, etc), but "bomb" commonly is just that (though I suppose it is also sometimes a cake). But the goal of naming like this is to choose something as clear as possible.
In our app.js
file let's add the following after the boardSize
is declared but before the board
is defined:
const bombCount = 20;
const validCount = boardSize - bombCount;
const squareValues = [];
for (let i = 0; i < boardSize; i++) {
if (i < bombCount) {
squareValues.push("bomb");
} else {
squareValues.push("valid");
}
}
Now we have an array of 20 bombs and 80 valids. We'll randomize that later so that the board is not just bombs at the top. But for now, let's use this array to create the board. So let's change our loop to live within a function (with a clear name) and use that array:
const createBoard = () => {
for (let value of squareValues) {
const clickedClass = "clicked";
const square = document.createElement("p");
square.innerHTML = value === "bomb" ? "💣" : "😀";
square.classList.add(value);
square.addEventListener("click", (e) => {
if (!square.classList.contains(clickedClass)) {
square.classList.add(clickedClass);
}
});
board.appendChild(square);
}
};
createBoard();
Note: If you're not familiar with for...of loops, they are very useful for iterating over every value in an array. Another option could be using squareValues.forEach()
, but in this case I prefer how the for...of loop looks.
Now when we refresh and check, we should see the 20 bomb icons in the top two rows of the board and the remaining of the board should be smiley faces.

Randomize the board when building
Our minesweeper game is going to get very stale very fast if the board is always populated with all the bombs up at the very top. So let's randomize the array we are building the board from each time we build the board.
Let's make a shuffleValues
function and call it on the first line inside the createBoard
function.
const shuffleValues = () => {
for (let i = 0; i < squareValues.length; i++) {
const randomIndex = Math.floor(Math.random() * squareValues.length);
let temp = squareValues[i];
squareValues[i] = squareValues[randomIndex];
squareValues[randomIndex] = temp;
}
};
This is a very simple method of scrambling our array, but it is pretty sufficient. Every element will switch with a random element, which could mean that an element will switch with itself, but that's okay since it's unlikely all 100 will switch with just themselves.
There's two steps to this function, for every element:
- Choose a random index
- Switch the element's value with the value at that index
Note: Instead of the temp switch method, another option with ES6 is to use destructuring: [squareValues[i], squareValues[randomIndex]] = [squareValues[randomIndex], squareValues[i]]
to switch them in a single line. Both are valid, though neither are immediately better than the other so choose accordingly.
Refresh and check and now our values should be randomized for every time we refresh the page.

Part 2 out now! Part 3 coming soon!
Check out part 2 to build out most of the gameplay. Add the